Registering
-----------
The idea is that game servers push their server info to the
masterservers every 15 seconds or when the server info changes, but not
more than once per second.
The game servers do not support the old registering protocol anymore,
the backward compatibility is handled by the masterserver.
The register call is a HTTP POST to a URL like
`https://master1.ddnet.tw/ddnet/15/register` and looks like this:
```json
POST /ddnet/15/register HTTP/1.1
Address: tw-0.6+udp://connecting-address.invalid:8303
Secret: 81fa3955-6f83-4290-818d-31c0906b1118
Challenge-Secret: 81fa3955-6f83-4290-818d-31c0906b1118:tw0.6/ipv6
Info-Serial: 0
{
"max_clients": 64,
"max_players": 64,
"passworded": false,
"game_type": "TestDDraceNetwork",
"name": "My DDNet server",
"map": {
"name": "dm1",
"sha256": "0b0c481d77519c32fbe85624ef16ec0fa9991aec7367ad538bd280f28d8c26cf",
"size": 5805
},
"version": "0.6.4, 16.0.3",
"clients": []
}
```
The `Address` header declares that the server wants to register itself as
a `tw-0.6+udp` server, i.e. a server speaking a Teeworlds-0.6-compatible
protocol.
The free-form `Secret` header is used as a server identity, the server
list will be deduplicated via this secret.
The free-form `Challenge-Secret` is sent back via UDP for a port forward
check. This might have security implications as the masterserver can be
asked to send a UDP packet containing some user-controlled bytes. This
is somewhat mitigated by the fact that it can only go to an
attacker-controlled IP address.
The `Info-Serial` header is an integer field that should increase each
time the server info (in the body) changes. The masterserver uses that
field to ensure that it doesn't use old server infos.
The body is a free-form JSON object set by the game server. It should
contain certain keys in the correct form to be accepted by clients. The
body is optional if the masterserver already confirmed the reception of
the info with the given `Info-Serial`.
Not shown in this payload is the `Connless-Token` header that is used
for Teeworlds 0.7 style communication.
Also not shown is the `Challenge-Token` that should be included once the
server receives the challenge token via UDP.
The masterserver responds with a `200 OK` with a body like this:
```
{"status":"success"}
```
The `status` field can be `success` if the server was successfully
registered on the masterserver, `need_challenge` if the masterserver
wants the correct `Challenge-Token` header before the register process
is successful, `need_info` if the server sent an empty body but the
masterserver doesn't actually know the server info.
It can also be `error` if the request was malformed, only in this case
an HTTP status code except `200 OK` is sent.
Synchronization
---------------
The masterserver keeps state and outputs JSON files every second.
```json
{
"servers": [
{
"addresses": [
"tw-0.6+udp://127.0.0.1:8303",
"tw-0.6+udp://[::1]:8303"
],
"info_serial": 0,
"info": {
"max_clients": 64,
"max_players": 64,
"passworded": false,
"game_type": "TestDDraceNetwork",
"name": "My DDNet server",
"map": {
"name": "dm1",
"sha256": "0b0c481d77519c32fbe85624ef16ec0fa9991aec7367ad538bd280f28d8c26cf",
"size": 5805
},
"version": "0.6.4, 16.0.3",
"clients": []
}
}
]
}
```
`servers.json` (or configured by `--out`) is a server list that is
compatible with DDNet 15.5+ clients. It is a JSON object containing a
single key `servers` with a list of game servers. Each game server is
represented by a JSON object with an `addresses` key containing a list
of all known addresses of the server and an `info` key containing the
free-form server info sent by the game server. The free-form `info` JSON
object re-encoded by the master server and thus canonicalized and
stripped of any whitespace characters outside strings.
```json
{
"kind": "mastersrv",
"now": 1816002,
"secrets": {
"tw-0.6+udp://127.0.0.1:8303": {
"ping_time": 1811999,
"secret": "42d8f991-f2fa-46e5-a9ae-ebcc93846feb"
},
"tw-0.6+udp://[::1]:8303": {
"ping_time": 1811999,
"secret": "42d8f991-f2fa-46e5-a9ae-ebcc93846feb"
}
},
"servers": {
"42d8f991-f2fa-46e5-a9ae-ebcc93846feb": {
"info_serial": 0,
"info": {
"max_clients": 64,
"max_players": 64,
"passworded": false,
"game_type": "TestDDraceNetwork",
"name": "My DDNet server",
"map": {
"name": "dm1",
"sha256": "0b0c481d77519c32fbe85624ef16ec0fa9991aec7367ad538bd280f28d8c26cf",
"size": 5805
},
"version": "0.6.4, 16.0.3",
"clients": []
}
}
}
}
```
`--write-dump` outputs a JSON file compatible with `--read-dump-dir`,
this can be used to synchronize servers across different masterservers.
`--read-dump-dir` is also used to ingest servers from the backward
compatibility layer that pings each server for their server info using
the old protocol.
The `kind` field describe that this is `mastersrv` output and not from a
`backcompat`. This is used for prioritizing `mastersrv` information over
`backcompat` information.
The `now` field contains an integer describing the current time in
milliseconds relative an unspecified epoch that is fixed for each JSON
file. This is done instead of using the current time as the epoch for
better compression of non-changing data.
`secrets` is a map from each server address and to a JSON object
containing the last ping time (`ping_time`) in milliseconds relative to
the same epoch as before, and the server secret (`secret`) that is used
to unify server infos from different addresses of the same logical
server.
`servers` is a map from the aforementioned `secret`s to the
corresponding `info_serial` and `info`.
```json
[
"tw-0.6+udp://127.0.0.1:8303",
"tw-0.6+udp://[::1]:8303"
]
```
`--write-addresses` outputs a JSON file containing all addresses
corresponding to servers that are registered to HTTP masterservers. It
does not contain the servers that are obtained via backward
compatibility measures.
This file can be used by an old-style masterserver to also list
new-style servers without the game servers having to register there.
An implementation of this can be found at
https://github.com/heinrich5991/teeworlds/tree/mastersrv_6_backcompat
for Teeworlds 0.5/0.6 masterservers and at
https://github.com/heinrich5991/teeworlds/tree/mastersrv_7_backcompat
for Teeworlds 0.7 masterservers.
All these JSON files can be sent over the network in an efficient way
using https://github.com/heinrich5991/twmaster-collect. It establishes a
zstd-compressed TCP connection authenticated by a string token that is
sent in plain-text. It watches the specified file and transmits it every
time it changes. Due to the zstd-compression, the data sent over the
network is similar to the size of a diff.
Implementation
--------------
The masterserver implementation was done in Rust.
The current gameserver register implementation doesn't support more than
one masterserver for registering.
These diagnostics are supposed to guide the user to problem resolution.
They're displayed if no packet is received from the server within one
second of connecting.
No message if we don't have STUN servers.
"Trying to determine UDP connectivity..." if no answer has been received
from the STUN server yet and it hasn't timed out yet.
"UDP seems to be filtered." if the STUN request has timed out.
"UDP and TCP IP addresses seem to be different. Try disabling VPN,
proxy or network accelerators." if the STUN request has returned an IP
address different from the one obtained via HTTP from info2.ddnet.tw.
"No answer from server yet." otherwise, if the STUN request has returned
no interesting data, indicating that it's likely the game server's
fault.
5085: New DDRace HUD r=def- a=C0D3D3V
If you want to test this PR, you have to test it on a Server that includes this PR too.
Textures are made by Ravie
Here a showcase video with most parts shown: https://youtu.be/gPTVj-s3pgc
Added to the new HUD
- A display of the weapons available to the player
- The weapon the player is carrying is highlighted
- Indicators for the special abilities of the player (Endless Hook, Endless Jumps, Jetpack, Teleport Weapons)
- Indicators for abilities taken away from the player (Deep/Life Freeze, No Hook, No Weapons collision, No Collision)
- Control indicators for dummy controls (dummy hammer, dummy copy) (bottom right)
- Jump indicator (max 10 jumps ar displayed, and greyed out as soon as a jump is used)
- Ninja status bar that indicates how long a player is capable of using ninja (next to the ninja sword)
- Freeze status bar that indicates the thawing time of a player (below player)
- Movement Information can be displayed in a clean way above the mini score HUD (Position, Speed, Target Angle)
- Indicator if you are in practice mode
The complete HUD also works for players you spectate
I Added a new NetObj since the predicted values are not perfect and would make the display of the information a lot less good: DDNetCharacterDisplayInfo that contains the following information
```
NetIntRange("m_JumpedTotal", -2, 255),
NetTick("m_NinjaActivationTick"),
NetTick("m_FreezeTick"),
NetBool("m_IsInFreeze"),
NetBool("m_IsInPracticeMode"),
NetIntAny("m_TargetX"), # used for the Movement Information display
NetIntAny("m_TargetY"),
NetIntAny("m_RampValue"),
```
So if someone has an idea what data we could also need in the client for making the display more nice, now is the right moment to add more data to this network object.
A few screenshots:
Assets Tab:
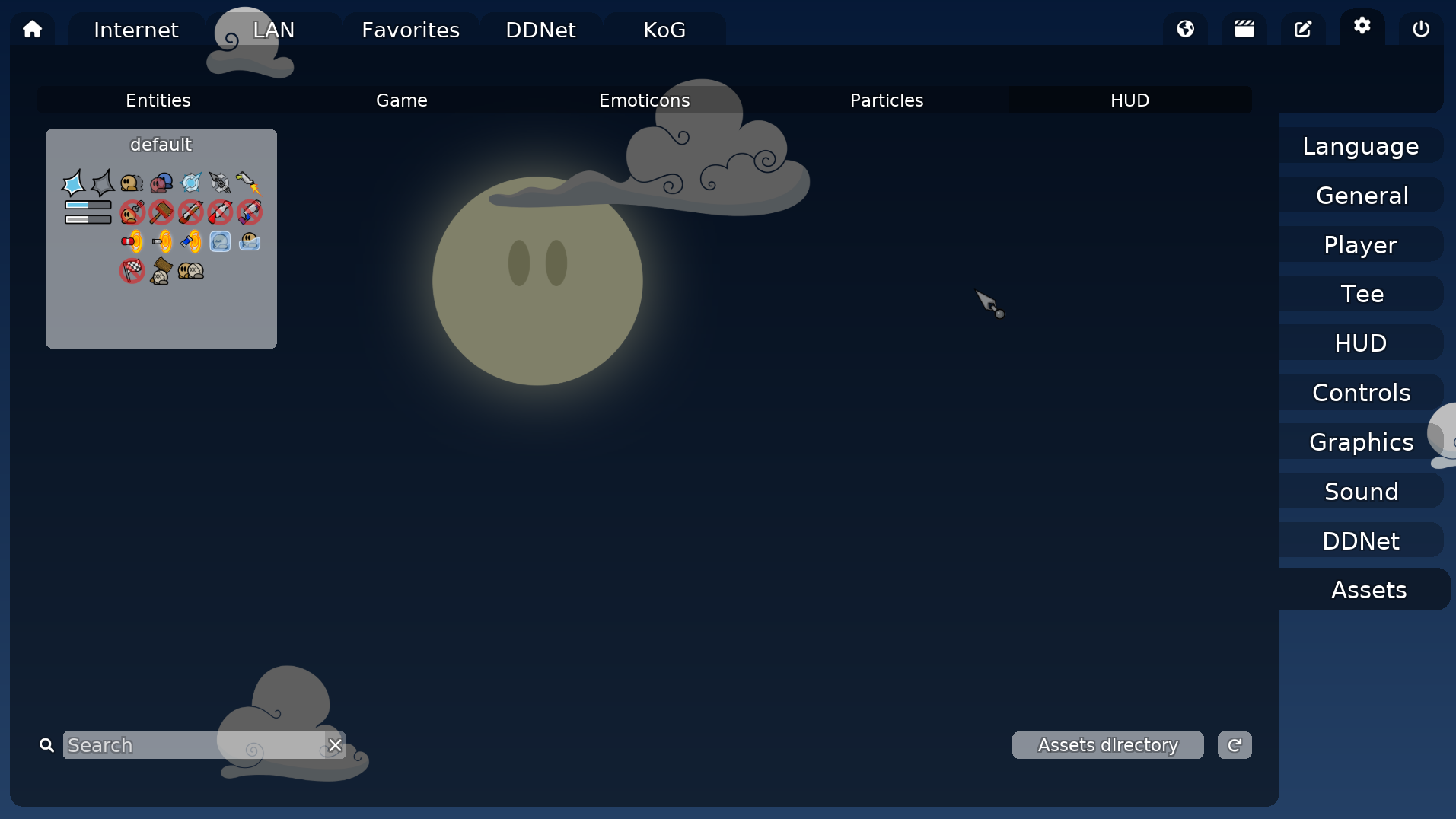
HUD Settings:
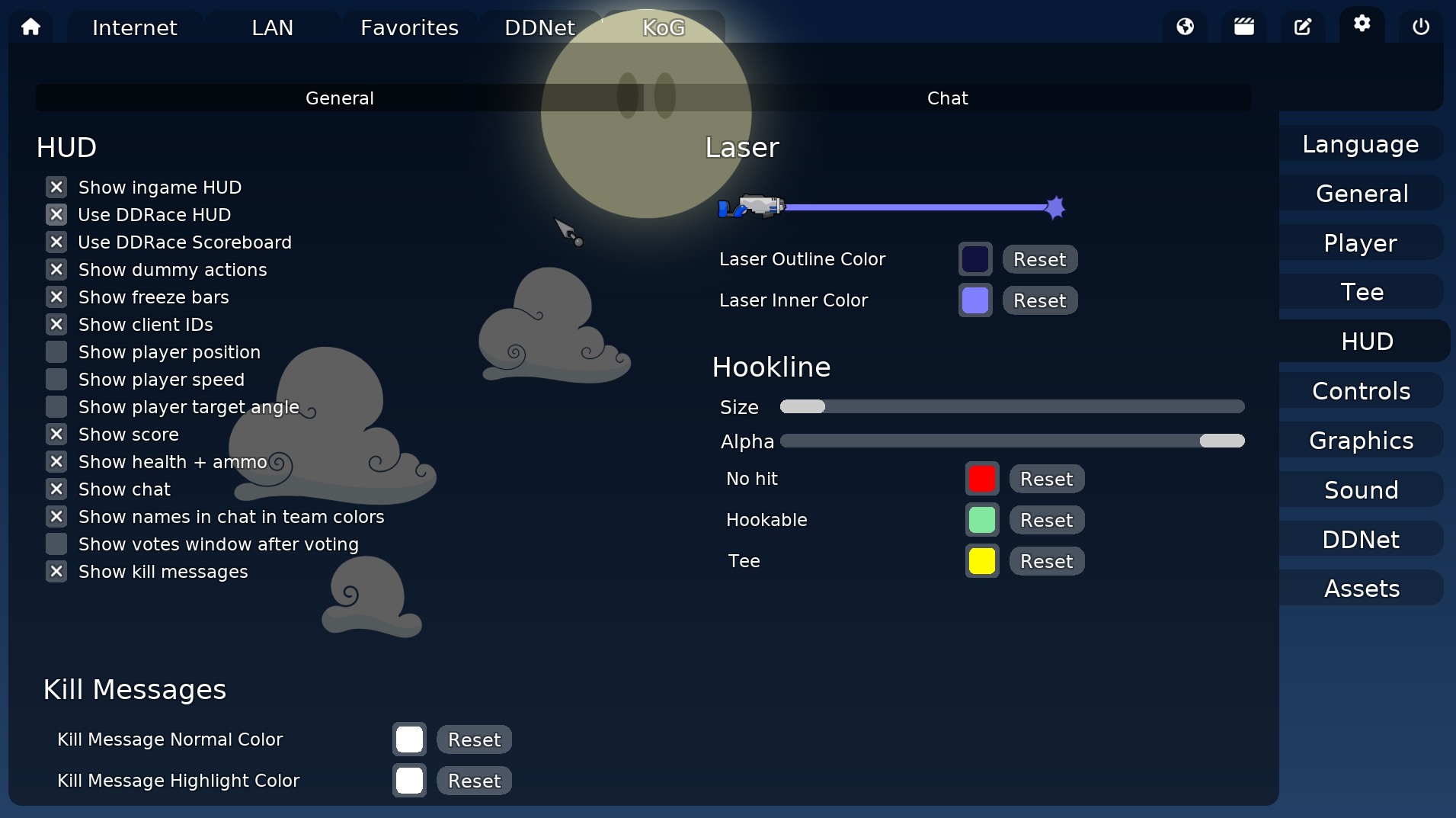
Mini Debug HUD:
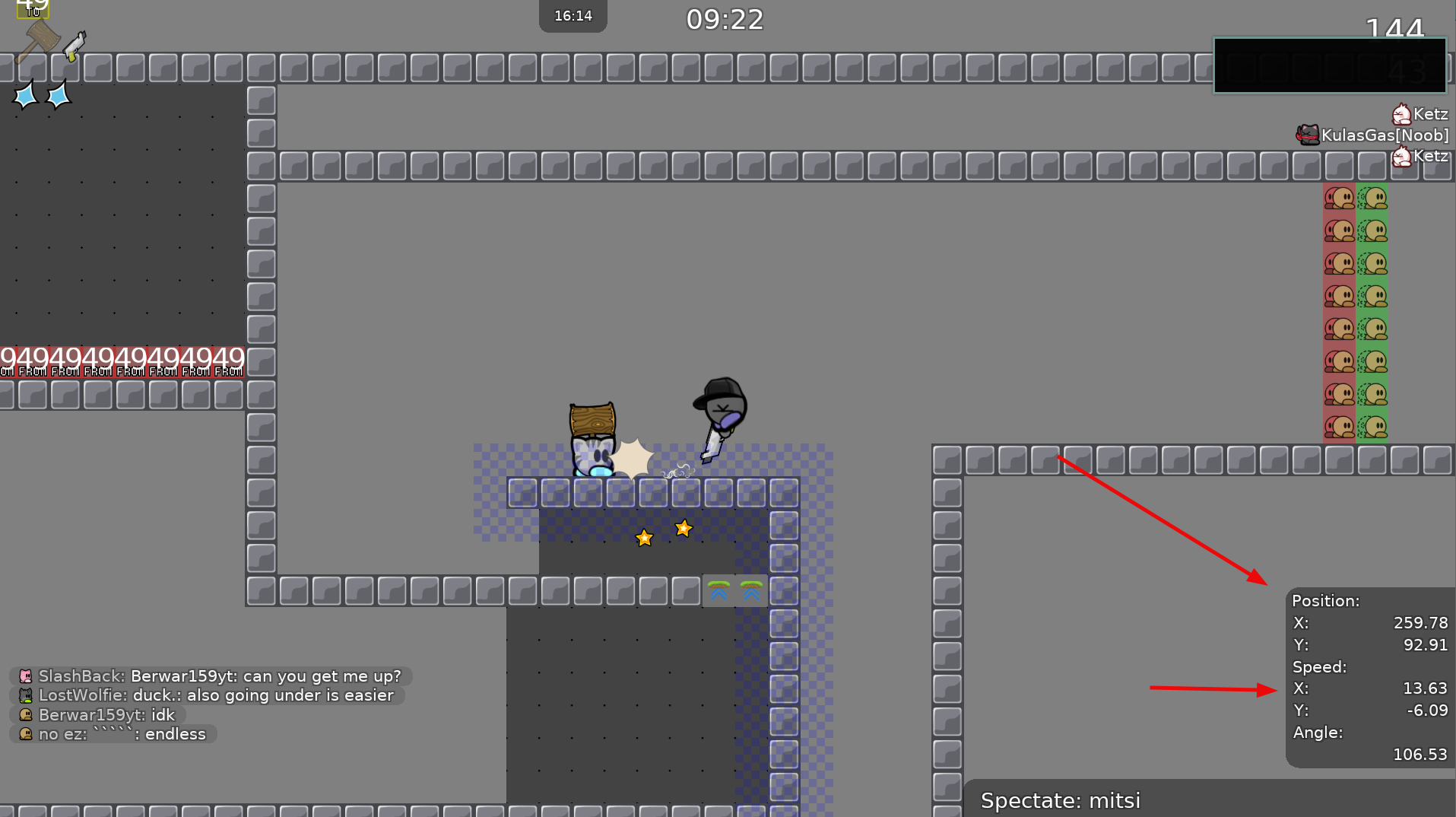
Speed.X is calculated using the players ramp vaule
## Checklist
- [x] Tested the change ingame
- [x] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [x] Considered possible null pointers and out of bounds array indexing
- [x] Changed no physics that affect existing maps
- [x] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: c0d3d3v <c0d3d3v@mag-keinen-spam.de>
Co-authored-by: Jupeyy <jupjopjap@gmail.com>
* master: (87 commits)
Remove base/tl/string.h
Replace remaining usage of base/tl/string with std::string
Remove unused includes of base/tl/string.h
Store localized strings in a CHeap instead of using tl/string.h
Mark methods as const
Add CHeap::StoreString method
Rules are chat responses too
Add margins to demo slice popup, decrease error font size, UI scaling
Remove redundant parameters which are overridden later
Use Margin instead of both VMargin and HMargin
Move variable declaration
Only output messages intended for chat to the user of a chat command
Remove unused chat response variables
Don't print the first "Waiting for score threads to complete"
fix usage of undefined behavior for default eyes
remove duplicate HOOK_RETRACTED assignment
do not send swap request notification to complete team 0
make swap messages more personal
Move ninja shield to other position (fixes#5047)
do not release the hooks if you swap
...
4845: Enable -Wshadow=local r=Jupeyy a=def-
> Warn when a local variable shadows another local variable or parameter.
Found one actual bug in graphics_threaded.cpp
Should reduce confusion in the future when reading source code
<!-- What is the motivation for the changes of this pull request -->
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: def <dennis@felsin9.de>
> Warn when a local variable shadows another local variable or parameter.
Found one actual bug in graphics_threaded.cpp
Should reduce confusion in the future when reading source code
4625: Use ETextAlignment enum for UI r=def- a=ChillerDragon
Replaces the magic numbers -1/0/1 for left/center/right
Based on the work done in upstream:
`@cinaera` added alignment enums
04ee8b20a1
`@TsFreddie` renamed them
67651e8122
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: ChillerDragon <ChillerDragon@gmail.com>
Replaces the magic numbers -1/0/1 for left/center/right
Based on the work done in upstream:
@cinaera added alignment enums
04ee8b20a1
@TsFreddie renamed them
67651e8122
like ctrl-f / cmd-f
On macOS all these system shortcuts are done with cmd while on Windows
ctrl is used. Support both now
Added support for cmd key as modifier for binds
3970: Fix pressing Home or End in server address field changes the selected server r=Learath2 a=BloodWod-513
<!-- What is the motivation for the changes of this pull request -->
I think it should fix#3964.
# Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [ ] Considered possible null pointers and out of bounds array indexing
- [x] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: BloodWod <dayn_2013@mail.ru>
3952: Remove global components and global gameclient instance r=heinrich5991 a=Jupeyy
Prevents global constructors/destructors and is a bit of cleanup
would also fix the issue from #3948
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [x] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Jupeyy <jupjopjap@gmail.com>
When a player wants to switch servers in-game, but needs to confirm the
action because of cl_confirm_disconnect, the game used to not actually
connect to the new server.
This adds a way to still connect to that server, after the player
confirms.
Signed-off-by: Sefa Eyeoglu <contact@scrumplex.net>
3304: Improve list box behaviour, make it more consistent r=heinrich5991 a=def-
Make keys work after searching in vote menu
Unify some duplicated code
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [x] Considered possible null pointers and out of bounds array indexing
- [x] Changed no physics that affect existing maps
- [x] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
3310: Found some more times with mins, use hours for consistency r=heinrich5991 a=def-
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test if it works standalone, system.c especially
- [x] Considered possible null pointers and out of bounds array indexing
- [x] Changed no physics that affect existing maps
- [x] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: def <dennis@felsin9.de>