Optimize MOTD rendering by caching the round rect and the text.
Align font and rect sizes so exactly 24 lines of text fit in the MOTD rect with margins on all sizes.
6372: Check in CI that no C standard headers are used, replace all usages of C standard headers with C++ headers, replace usages of C math functions r=def- a=Robyt3
Closes#6334.
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
Replacing the C standard headers with the C++ standard headers causes various `error: call to 'floor' promotes float to double [performance-type-promotion-in-math-fn,-warnings-as-errors]`, which are fixed by using the C++ std math functions or our own math functions instead of the C math functions.
- Use `absolute` instead of `abs` and `fabs`.
- Use `std::floor` instead of `floor` and `floorf`.
- Use `std::ceil` instead of `ceil`, `ceilf` and `round_ceil`.
- Use `std::round` instead of `round` and `roundf`.
- Use `std::sin` instead of `sin` and `sinf`.
- Use `std::asin` instead of `asin` and `asinf`.
- Use `std::cos` instead of `cos` and `cosf`.
- Use `std::acos` instead of `acos` and `acosf`.
- Use `std::tan` instead of `tan` and `tanf`.
- Use `std::atan` instead of `atan` and `atanf`.
- Use `std::pow` instead of `pow` and `powf`.
- Use `std::log` instead of `log` and `logf`.
- Use `std::log2` instead of `log2` and `log2f`.
- Use `std::log10` instead of `log10` and `log10f`.
- Use `std::pow` instead of `pow` and `powf`.
- Use `std::sqrt` instead of `sqrt` and `sqrtf`.
- Use `std::fmod` instead of `fmod` and `fmodf`.
- Use `direction(Angle)` instead of `vec2(std::cos(Angle), std::sin(Angle))`.
- Use `length(vec2(x, y))` instead of `std::sqrt(x * x + y * y)`.
- Remove unused `NormalizeAngular` and `AngularDistance` functions.
The graphics are not initialised when `RebuildChat` is called by `ConchainChatOld`, which causes the client to crash if `cl_chat_old` is present in the user's config or used as a command line argument.
6364: Fix incorrect text wrapping in ingame server info r=def- a=Robyt3
The maximum width for the server info and game info text was too small, which previously didn't matter, because the manual newlines broke text wrapping in this case, but with the fix from #6353 this now causes the text to break after the colons already.
This is fixed by using the maximum available width for the text instead of a too small magic number (`250.0f`).
For completeness, the maximum width for the title texts is unset (`-1.0f`), because they should never wrap, which is consistent with the MOTD title text.
Before:
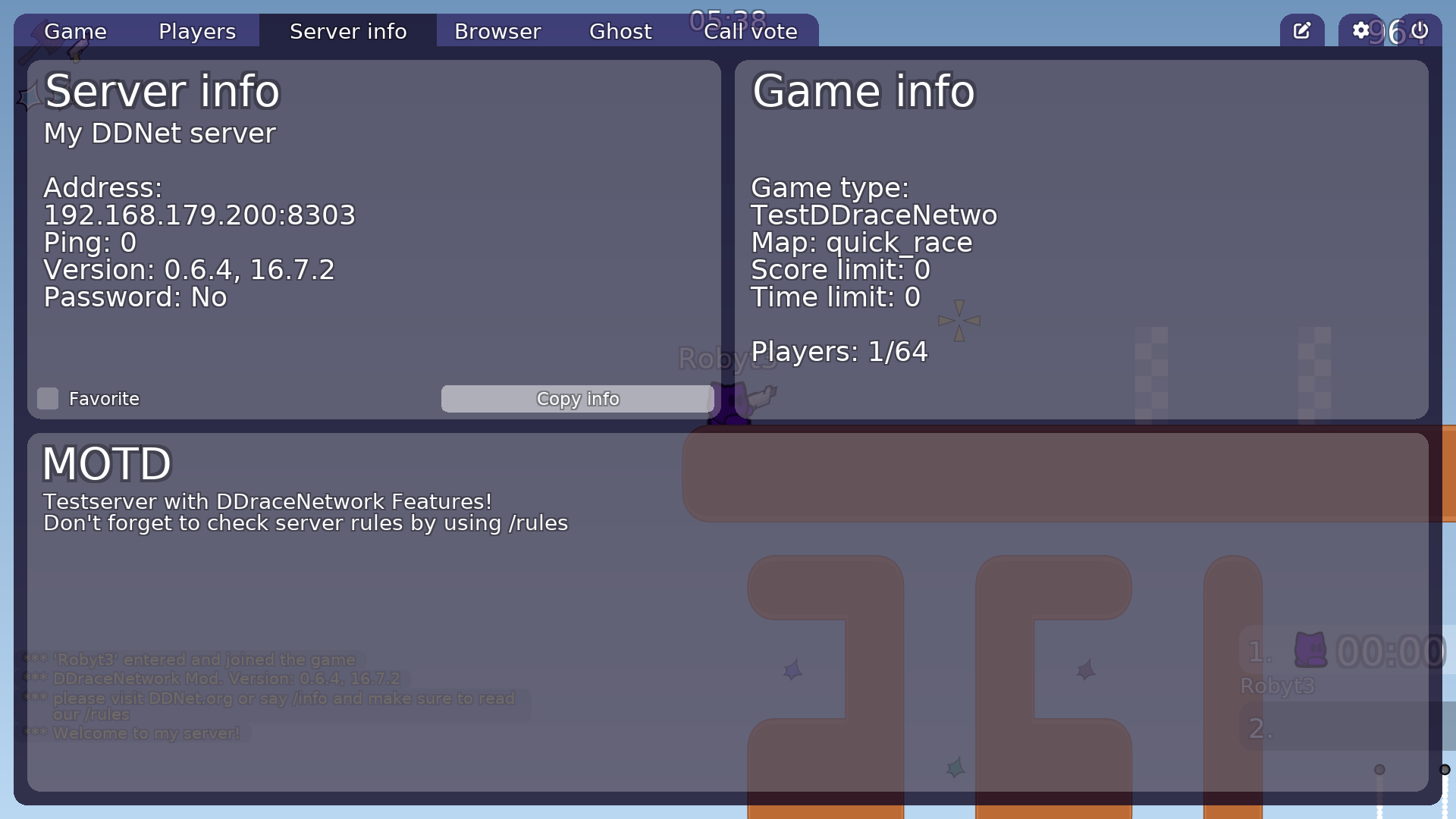
After:
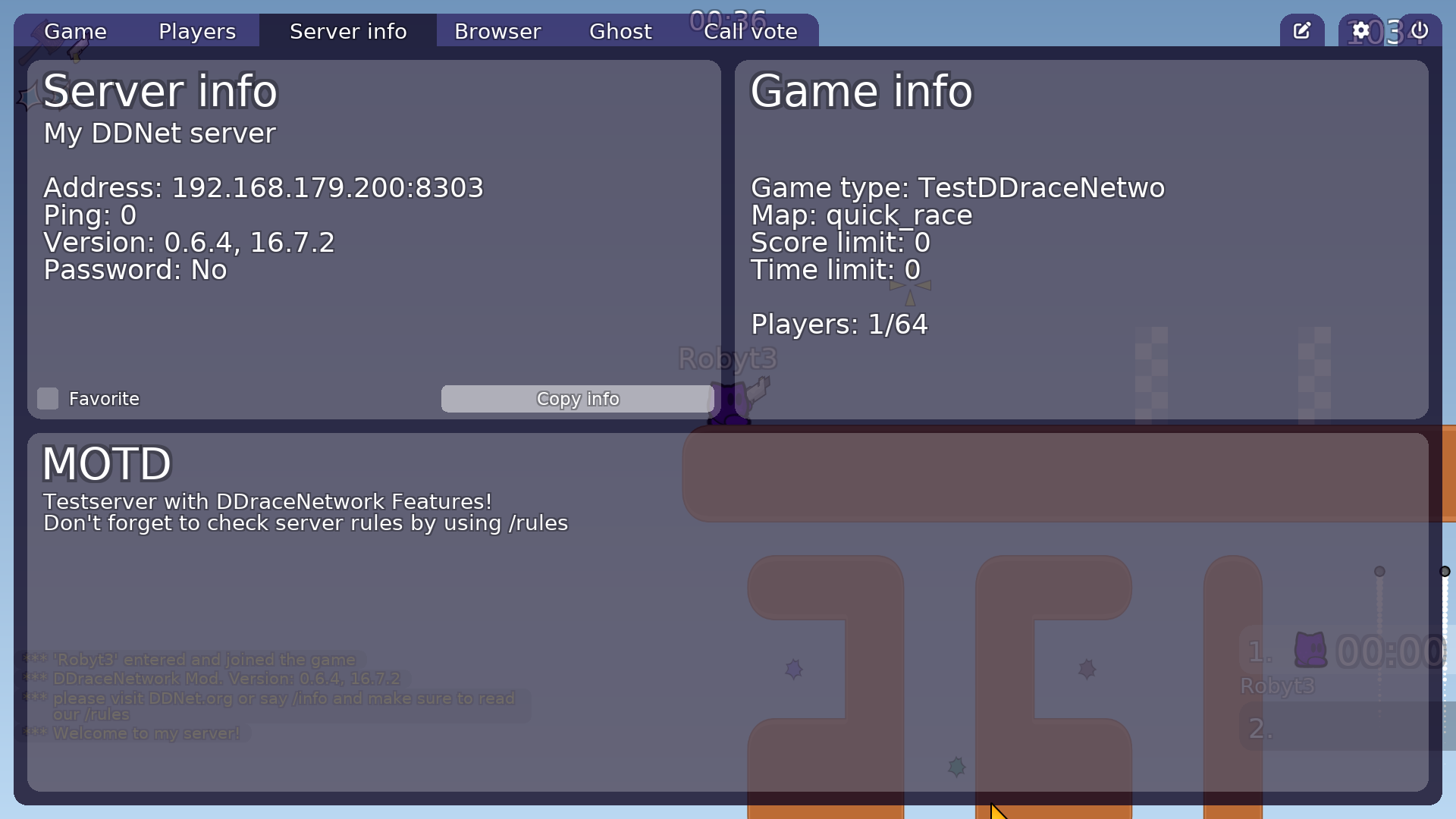
## Checklist
- [X] Tested the change ingame
- [X] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
Remember the maximum animation time when initiating a smooth scroll, so the smooth scrolling does not change erratically when `ui_smooth_scroll_time` is changed while smooth scrolling is in progress.
The maximum width for the server info and game info text was too small, which previously didn't matter, because the manual newlines broke text wrapping in this case, but with the fix from #6353 this now causes the text to break after the colons already.
This is fixed by using the maximum available width for the text instead of a too small magic number (`250.0f`).
For completeness, the maximum width for the title texts is unset (`-1.0f`), because they should never wrap, which is consistent with the MOTD title text.
6351: Fix client crash when echoing client message to chat, use em dash for client messages in chat like on upstream r=def- a=Robyt3
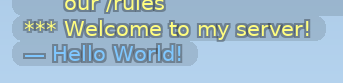
## Checklist
- [X] Tested the change ingame
- [X] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
Align the color picker selection and reset buttons on the right side instead of the left side dependent on the label width.
Closes#6237.
The `UseCheckBox` argument is removed and instead `pCheckBoxValue != nullptr` is used.
The client crashes when printing a client message to the chat from a config file or from the command line, as the graphics have not been initialized at that point.
Closes#6350.
The `RecreateTextContainer` function calls `DeleteTextContainer` and then `CreateTextContainer`.
The arguments of `RecreateTextContainer` and `RecreateTextContainerSoft` are reordered so all functions take the text container as their first argument.
Reduce duplicate code and improve correctness by passing indices of quad, buffer and text containers by reference and always setting them to `-1` after they are deleted.
Also check if index is `-1` before trying to delete it to reduce duplicate code when calling the methods.
6328: Remove `bytes_be_to_int` and `int_to_bytes_be`, static assert size of `int` and `unsigned`, refactoring r=heinrich5991 a=Robyt3
Supersedes #6263.
## Checklist
- [X] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
Use `bytes_be_to_uint` and `uint_to_bytes_be` instead.
As casting between `int` and `unsigned` preserves the bit representation of the value, it's not necessary to apply additional tricks to convert between `char` arrays and `int`.
6293: rewrite int64_t to bitset for clients mask r=Robyt3 a=0xfaulty
Alternative version for PR [#6292](https://github.com/ddnet/ddnet/pull/6292) with bitset used.
I did the naming as I would like, but I can change it if there is a more suitable one, typedef is just for shortening, can be removed.
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Valentin Bashkirov <v.bashkirov@dev.tassta.com>
Co-authored-by: Valentin Bashkirov <valenteen3d@ya.ru>
Using `str_format` without format arguments is equivalent to `str_copy`, but using the latter is more efficient and readable.
As `str_format` also returns the result `str_utf8_fix_truncation`, i.e. the potentially truncated string length, the return value is also added to `str_copy` so existing invocations don't need to be adjusted.
Alternative to #6294
The only remaining problems are:
/home/deen/git/ddnet/src/engine/client/backend/glsl_shader_compiler.cpp:22:26: warning: unnecessary temporary object created while calling emplace_back [modernize-use-emplace]
m_vDefines.emplace_back(SGLSLCompilerDefine(DefineName, DefineValue));
^~~~~~~~~~~~~~~~~~~~ ~
This variable adjusts the time for smooth scrolling of all scrollregions and listboxes in the menus and editor.
The value `0` disables smooth scrolling entirely.
6249: Fix DoLabelStreamed by using offsets, instead of rebuilding when x,y changes r=Robyt3 a=Jupeyy
<!-- What is the motivation for the changes of this pull request? -->
<!-- Note that builds and other checks will be run for your change. Don't feel intimidated by failures in some of the checks. If you can't resolve them yourself, experienced devs can also resolve them before merging your pull request. -->
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Jupeyy <jupjopjap@gmail.com>
It was no longer possible to navigate listboxes (e.g. the server browser) by using keyboard hotkeys when no item is currently selected due to a regression from #6240.
Additionally, it was not possible even before #6240 to start navigating the server browser with the keyboard afer manually clearing the server address input, which is now possible as well.
6251: Always assume there could potentially be a skin in serverbrowser's pl… r=def- a=Jupeyy
…ayer list
<!-- What is the motivation for the changes of this pull request? -->
<!-- Note that builds and other checks will be run for your change. Don't feel intimidated by failures in some of the checks. If you can't resolve them yourself, experienced devs can also resolve them before merging your pull request. -->
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Jupeyy <jupjopjap@gmail.com>
The selected server browser entry was not being updated anymore when the server address input is changed manually by the user or when selecting an entry in the LAN server list and then switching back to the Internet list.
Regression from #6240.
Add "Copy info" buttons to server browser and ingame menu to copy the server info of the selected/current server to the clipboard.
The margins around the server browser details are improved.
Closes#5440.
Replace existing listbox implementations (`CMenus::UiDoListbox*` and `HandleListInputs` functions) with `CListBox` from upstream.
Reimplement additional feature that was already present in ddnet: page up/down, home and end key handling.
Affects the following lists:
- server browser
- server browser scoreboard
- server browser friends
- country / region selection popup (server browser filter)
- player skin list
- player country / region list
- theme list
- assets list
- graphics resolutions list
- dropdown menus (e.g. graphics fullscreen mode)
- ingame player list
- vote options list
- kick/specvote lists
- ghost list
- language list (in settings and in popup on first launch)
- demo browser
- editor file browser (saving, loading, adding images / sounds)
- The search / filename input is also improved so navigating a filtered list works correctly by porting the logic from upstream.
There are minor changes to the visual appearance of some lists, due to changed margins.
The vertical alignment of some list item texts is improved so the text is centered vertically.