If the player slots update the 0.7 clients have to be informed
about it. Otherwise the client can block the join button
if the outdated playerslots are filled already.
Add more efficient function for formatting integer values as strings.
A benchmark shows that using this function is significantly faster than using `str_format`. It is faster by a factor of 220 with Clang 15.0 O2 (https://quick-bench.com/q/BlNoLnlyqxipf4jvsFTUxKMHDJU) and by a factor of 11 with GCC 12.2 O2 (https://quick-bench.com/q/Fxf9lDCTqXBF4pIa_IyZ5R0IqYg).
This increases FPS in the editor by ~25% when many numbers are rendered for switch/tele/speedup/tune layers or with "Show Info" being enabled.
The additional static analysis for `std::to_chars` revealed that the wrong size was used in `CHud` for `aScoreTeam[TEAM_RED]` and `aScoreTeam[TEAM_BLUE]`.
This requires incrementing the macOS deployment target from 10.13 to 10.15.
vanilla 0.6 joins weren't recorded. Also after map change, for the
existing players these Join chunks were missing. Just add these
message for existing players now and add OnClientEngineJoin in all
code paths with clients connecting.
`stdout_output_level` for printing to stdout, `console_output_level` for
printing to local console and remote console and `loglevel` for the log
file.
Keep the old log level filters 0 for info and more severe, 1 for debug
and more severe and 2 for trace and more severe, introducing -1 for
warn, and -2 for error. -3 will show no log messages at all.
Use `std::vector` in cases where elements are only inserted at the end of the collection.
Use `std::deque` in cases where elements are only inserted/deleted at the beginning/end of the collection.
Use `std::list` in the remaining single case where elements are being removed from arbitrary positions and added at either the beginning or the end of the collection.
Adjust variables names. Don't use separate prefix for `std::deque`s and `std::list`s, as they are only used very rarely. Closes#6779.
Quit client and server if the configured bindaddr cannot be resolved.
Disable econ if configured bindaddr cannot be resolved.
To ensure that the configured bindaddr is not silently ignored.
The client measures the time difference between
ping send and ping reply receive.
Without MSGFLAG_FLUSH the server keeps the chunk
until the next flush which makes the ping dependend
on when the next flush happens.
6295: Implement FIFO on Windows using Named Pipes r=def- a=Robyt3
Reimplement the Linux FIFO file server and client controls on Windows by using Named Pipes.
The DDNet server/client acts as a named pipe server and receives messages.
Messages can be posted to the named pipe server by connecting to it as a client.
The named pipe client can for instance be controlled from the command line with PowerShell.
The PowerShell script `scripts/send_named_pipe.ps1` is added for this purpose.
For example the PowerShell command `./send_named_pipe.ps1 "testpipe" "echo a"` sends the command `echo a` to the pipe named `testpipe`.
Multiple commands can be sent at the same time by separating them with semicolons or newlines.
## Checklist
- [X] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
6215: Don't count (connecting) players for voting r=Robyt3 a=def-
<!-- What is the motivation for the changes of this pull request? -->
<!-- Note that builds and other checks will be run for your change. Don't feel intimidated by failures in some of the checks. If you can't resolve them yourself, experienced devs can also resolve them before merging your pull request. -->
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: def <dennis@felsin9.de>
Reimplement the Linux FIFO file server and client controls on Windows by using Named Pipes.
The DDNet server/client acts as a named pipe server and receives messages.
Messages can be posted to the named pipe server by connecting to it as a client.
The named pipe client can for instance be controlled from the command line with PowerShell.
The PowerShell script `scripts/send_named_pipe.ps1` is added for this purpose.
For example the PowerShell command `./send_named_pipe.ps1 "testpipe" "echo a"` sends the command `echo a` to the pipe named `testpipe`.
Multiple commands can be sent at the same time by separating them with semicolons or newlines.
Swap mathematical operations to avoid multiplication by `1000`. As `time_freq()` returns the nanoseconds in a second (1e9), first dividing this number by 1000 does not lose any precision.
Also ensure that `IntendedTick` is in the valid range of ticks.
```
src/engine/server/server.cpp:1585:64: runtime error: signed integer overflow: 24185120014282423 * 1000 cannot be represented in type 'long int'
0 0x55c165f220aa in CServer::ProcessClientPacket(CNetChunk*) src/engine/server/server.cpp:1579
1 0x55c165f3a8d3 in CServer::PumpNetwork(bool) src/engine/server/server.cpp:2383
2 0x55c165f51166 in CServer::Run() src/engine/server/server.cpp:2821
3 0x55c165eb37d0 in main src/engine/server/main.cpp:191
4 0x7f99e4c3ad8f in __libc_start_call_main ../sysdeps/nptl/libc_start_call_main.h:58
5 0x7f99e4c3ae3f in __libc_start_main_impl ../csu/libc-start.c:392
6 0x55c165e7ab64 in _start (build-asan/DDNet-Server+0xd7ab64)
```
6035: Fix various issues reported by cppcheck static analyser r=def- a=Robyt3
After generating `compile_commands.json` with cmake, I ran [cppcheck](https://cppcheck.sourceforge.io/) like this:
```
cppcheck --project=compile_commands.json -DWIN64 --suppressions-list=cppcheck.supp --enable=all 2>cppcheck.log
```
With these suppressions in `cppcheck.supp`:
```
cstyleCast
useStlAlgorithm
unusedFunction
variableScope
noExplicitConstructor
useInitializationList
noConstructor
uninitMemberVar
uninitMemberVarPrivate
uninitDerivedMemberVar
uninitStructMember
uninitvar
shadowFunction
memleakOnRealloc
internalAstError
virtualCallInConstructor
unknownMacro
noOperatorEq
noCopyConstructor
```
Many of these occur too often or are false positives.
Here is a list of all remaining non-suppressed issues reported by cppcheck: [cppcheck.log](https://github.com/ddnet/ddnet/files/9997663/cppcheck.log)
And here is a list of all remaining issues including the suppressed ones: [cppcheck_all.log](https://github.com/ddnet/ddnet/files/9997662/cppcheck_all.log)
I couldn't get cppcheck's command line argument to ignore the external folders to work correctly, so I manually removed those entries from the files.
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
According to cppcheck's `constVariable` error:
```
src\engine\client\backend\opengl\opengl_sl.cpp:74:43: style: Variable 'Define' can be declared as reference to const [constVariable]
for(CGLSLCompiler::SGLSLCompilerDefine &Define : pCompiler->m_vDefines)
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:2149:12: style: Variable 'GraphicThreadCommandBuffer' can be declared as reference to const [constVariable]
auto &GraphicThreadCommandBuffer = m_vvThreadDrawCommandBuffers[i + 1][m_CurImageIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:3192:9: style: Variable 'BufferObject' can be declared as reference to const [constVariable]
auto &BufferObject = m_vBufferObjects[BufferObjectIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:3200:10: style: Variable 'DescrSet' can be declared as reference to const [constVariable]
auto &DescrSet = m_vTextures[State.m_Texture].m_VKStandard3DTexturedDescrSet;
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:3810:13: style: Variable 'Mode' can be declared as reference to const [constVariable]
for(auto &Mode : vPresentModeList)
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:3818:13: style: Variable 'Mode' can be declared as reference to const [constVariable]
for(auto &Mode : vPresentModeList)
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6511:10: style: Variable 'DescrSet' can be declared as reference to const [constVariable]
auto &DescrSet = m_vTextures[pCommand->m_State.m_Texture].m_aVKStandardTexturedDescrSets[AddressModeIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6555:10: style: Variable 'DescrSet' can be declared as reference to const [constVariable]
auto &DescrSet = m_vTextures[pCommand->m_State.m_Texture].m_VKStandard3DTexturedDescrSet;
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6660:9: style: Variable 'MemBlock' can be declared as reference to const [constVariable]
auto &MemBlock = m_vBufferObjects[BufferIndex].m_BufferObject.m_Mem;
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6799:9: style: Variable 'BufferObject' can be declared as reference to const [constVariable]
auto &BufferObject = m_vBufferObjects[BufferObjectIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6808:10: style: Variable 'DescrSet' can be declared as reference to const [constVariable]
auto &DescrSet = m_vTextures[pCommand->m_State.m_Texture].m_aVKStandardTexturedDescrSets[AddressModeIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6902:9: style: Variable 'BufferObject' can be declared as reference to const [constVariable]
auto &BufferObject = m_vBufferObjects[BufferObjectIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6907:9: style: Variable 'TextTextureDescr' can be declared as reference to const [constVariable]
auto &TextTextureDescr = m_vTextures[pCommand->m_TextTextureIndex].m_VKTextDescrSet;
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6961:9: style: Variable 'BufferObject' can be declared as reference to const [constVariable]
auto &BufferObject = m_vBufferObjects[BufferObjectIndex];
^
src\engine\client\backend\vulkan\backend_vulkan.cpp:6970:10: style: Variable 'DescrSet' can be declared as reference to const [constVariable]
auto &DescrSet = m_vTextures[State.m_Texture].m_aVKStandardTexturedDescrSets[AddressModeIndex];
^
src\game\client\components\hud.cpp:178:8: style: Variable 'aFlagCarrier' can be declared as const array [constVariable]
int aFlagCarrier[2] = {
^
src\game\client\components\hud.cpp:519:16: style: Variable 's_aTextWidth' can be declared as const array [constVariable]
static float s_aTextWidth[5] = {s_TextWidth0, s_TextWidth00, s_TextWidth000, s_TextWidth0000, s_TextWidth00000};
^
src\game\client\components\killmessages.cpp:305:30: style: Variable 'Client' can be declared as reference to const [constVariable]
CGameClient::CClientData &Client = GameClient()->m_aClients[m_aKillmsgs[r].m_KillerID];
^
src\game\client\components\killmessages.cpp:314:30: style: Variable 'Client' can be declared as reference to const [constVariable]
CGameClient::CClientData &Client = GameClient()->m_aClients[m_aKillmsgs[r].m_VictimID];
^
src\game\client\components\menus_ingame.cpp:243:12: style: Variable 'pInfoByName' can be declared as reference to const [constVariable]
for(auto &pInfoByName : m_pClient->m_Snap.m_apInfoByName)
^
src\game\client\components\menus_ingame.cpp:530:12: style: Variable 'pInfoByName' can be declared as reference to const [constVariable]
for(auto &pInfoByName : m_pClient->m_Snap.m_apInfoByName)
^
src\game\client\components\players.cpp:767:44: style: Variable 'CharacterInfo' can be declared as reference to const [constVariable]
CGameClient::CSnapState::CCharacterInfo &CharacterInfo = m_pClient->m_Snap.m_aCharacters[i];
^
src\game\client\components\spectator.cpp:122:27: style: Variable 'Snap' can be declared as reference to const [constVariable]
CGameClient::CSnapState &Snap = pSelf->m_pClient->m_Snap;
^
src\game\client\components\spectator.cpp:221:12: style: Variable 'pInfo' can be declared as reference to const [constVariable]
for(auto &pInfo : m_pClient->m_Snap.m_apInfoByDDTeamName)
^
src\game\client\gameclient.cpp:2220:15: style: Variable 'OwnClientData' can be declared as reference to const [constVariable]
CClientData &OwnClientData = m_aClients[ownID];
^
src\game\client\gameclient.cpp:2227:16: style: Variable 'cData' can be declared as reference to const [constVariable]
CClientData &cData = m_aClients[i];
^
src\game\client\prediction\entities\character.cpp:397:11: style: Variable 'aSpreading' can be declared as const array [constVariable]
float aSpreading[] = {-0.185f, -0.070f, 0, 0.070f, 0.185f};
^
src\game\client\prediction\entities\laser.cpp:53:9: style: Variable 'HitPos' can be declared as reference to const [constVariable]
vec2 &HitPos = pHit->Core()->m_Pos;
^
src\game\editor\auto_map.cpp:507:18: style: Variable 'Index' can be declared as reference to const [constVariable]
for(auto &Index : pRule->m_vIndexList)
^
src\game\editor\auto_map.cpp:518:18: style: Variable 'Index' can be declared as reference to const [constVariable]
for(auto &Index : pRule->m_vIndexList)
^
src\game\editor\editor.cpp:118:12: style: Variable 'Item' can be declared as reference to const [constVariable]
for(auto &Item : vList)
^
src\game\editor\editor.cpp:2983:11: style: Variable 'aAspects' can be declared as const array [constVariable]
float aAspects[] = {4.0f / 3.0f, 16.0f / 10.0f, 5.0f / 4.0f, 16.0f / 9.0f};
^
src\game\editor\editor.cpp:3141:15: style: Variable 's_aShift' can be declared as const array [constVariable]
static int s_aShift[] = {24, 16, 8, 0};
^
src\engine\server\server.cpp:2807:14: style: Variable 'Client' can be declared as reference to const [constVariable]
for(auto &Client : m_aClients)
^
src\engine\server\sql_string_helpers.cpp:51:6: style: Variable 'aTimes' can be declared as const array [constVariable]
int aTimes[7] =
^
src\test\secure_random.cpp:24:6: style: Variable 'BOUNDS' can be declared as const array [constVariable]
int BOUNDS[] = {2, 3, 4, 5, 10, 100, 127, 128, 129};
^
```
According to cppchecker's `arrayIndexOutOfBoundsCond` error:
```
src\engine\server\server.cpp:117:19: warning: Either the condition 'ID<0' is redundant or the array 'm_aIDs[32768]' is accessed at index 32768, which is out of bounds. [arrayIndexOutOfBoundsCond]
dbg_assert(m_aIDs[ID].m_State == ID_ALLOCATED, "id is not allocated");
^
src\engine\server\server.cpp:115:8: note: Assuming that condition 'ID<0' is not redundant
if(ID < 0)
^
src\engine\server\server.cpp:117:19: note: Array index out of bounds
dbg_assert(m_aIDs[ID].m_State == ID_ALLOCATED, "id is not allocated");
^
src\engine\server\server.cpp:120:8: warning: Either the condition 'ID<0' is redundant or the array 'm_aIDs[32768]' is accessed at index 32768, which is out of bounds. [arrayIndexOutOfBoundsCond]
m_aIDs[ID].m_State = ID_TIMED;
^
src\engine\server\server.cpp:115:8: note: Assuming that condition 'ID<0' is not redundant
if(ID < 0)
^
src\engine\server\server.cpp:120:8: note: Array index out of bounds
m_aIDs[ID].m_State = ID_TIMED;
^
src\engine\server\server.cpp:121:8: warning: Either the condition 'ID<0' is redundant or the array 'm_aIDs[32768]' is accessed at index 32768, which is out of bounds. [arrayIndexOutOfBoundsCond]
m_aIDs[ID].m_Timeout = time_get() + time_freq() * 5;
^
src\engine\server\server.cpp:115:8: note: Assuming that condition 'ID<0' is not redundant
if(ID < 0)
^
src\engine\server\server.cpp:121:8: note: Array index out of bounds
m_aIDs[ID].m_Timeout = time_get() + time_freq() * 5;
^
src\engine\server\server.cpp:122:8: warning: Either the condition 'ID<0' is redundant or the array 'm_aIDs[32768]' is accessed at index 32768, which is out of bounds. [arrayIndexOutOfBoundsCond]
m_aIDs[ID].m_Next = -1;
^
src\engine\server\server.cpp:115:8: note: Assuming that condition 'ID<0' is not redundant
if(ID < 0)
^
src\engine\server\server.cpp:122:8: note: Array index out of bounds
m_aIDs[ID].m_Next = -1;
^
```
According to cppcheck's `badBitmaskCheck` error:
```
src\engine\client\client.cpp:422:26: style: Operator '|' with one operand equal to zero is redundant. [badBitmaskCheck]
Packer.AddInt((0 << 1) | (pMsg->m_System ? 1 : 0)); // NETMSG_EX, NETMSGTYPE_EX
^
src\engine\shared\snapshot.cpp:40:45: style: Operator '|' with one operand equal to zero is redundant. [badBitmaskCheck]
int TypeItemIndex = GetItemIndex((0 << 16) | InternalType); // NETOBJTYPE_EX
^
src\engine\server\server.cpp:777:26: style: Operator '|' with one operand equal to zero is redundant. [badBitmaskCheck]
Packer.AddInt((0 << 1) | (pMsg->m_System ? 1 : 0)); // NETMSG_EX, NETMSGTYPE_EX
^
```
The types are translated to `TYPE_ALL`/`TYPE_SAVE` respectively if a given path is relative and to `TYPE_ABSOLUTE` if a path is absolute.
These types are only supported with the `OpenFile`, `ReadFile`, `ReadFileStr` and `GetCompletePath` methods.
This reduces duplicate code when calling the methods.
5599: Add support for Rust code in DDNet r=def- a=heinrich5991
The glue is done using the [cxx crate](https://cxx.rs/) on the Rust side.
As a proof-of-concept, only a small console command (`rust_version`) printing the currently used Rust version was added.
You can generate and open the Rust documentation using `DDNET_TEST_NO_LINK=1 cargo doc --open`.
You can run the Rust tests using `cmake --build <build dir> --target run_rust_tests`, they're automatically included in the `run_tests` target as well.
Rust tests don't work on Windows in debug mode on Windows because Rust cannot currently link with the debug version of the C stdlib on Windows: https://github.com/rust-lang/rust/issues/39016.
---
The stuff in `src/rust-bridge` is generated using
```
cxxbridge src/engine/shared/rust_version.rs --output src/rust-bridge/engine/shared/rust_version.cpp --output src/rust-bridge/engine/shared/rust_version.h
cxxbridge src/engine/console.rs --output src/rust-bridge/cpp/console.cpp --output src/rust-bridge/cpp/console.h
```
Co-authored-by: heinrich5991 <heinrich5991@gmail.com>
The glue is done using the [cxx crate](https://cxx.rs/) on the Rust
side.
As a proof-of-concept, only a small console command (`rust_version`)
printing the currently used Rust version was added.
You can generate and open the Rust documentation using
`DDNET_TEST_NO_LINK=1 cargo doc --open`.
You can run the Rust tests using `cmake --build <build dir> --target
run_rust_tests`, they're automatically included in the `run_tests`
target as well.
Rust tests don't work on Windows in debug mode on Windows because Rust
cannot currently link with the debug version of the C stdlib on Windows:
https://github.com/rust-lang/rust/issues/39016.
---
The stuff in `src/rust-bridge` is generated using
```
cxxbridge src/engine/shared/rust_version.rs --output src/rust-bridge/engine/shared/rust_version.cpp --output src/rust-bridge/engine/shared/rust_version.h
cxxbridge src/engine/console.rs --output src/rust-bridge/cpp/console.cpp --output src/rust-bridge/cpp/console.h
```
5848: Remove cl_http_map_download r=heinrich5991 a=def-
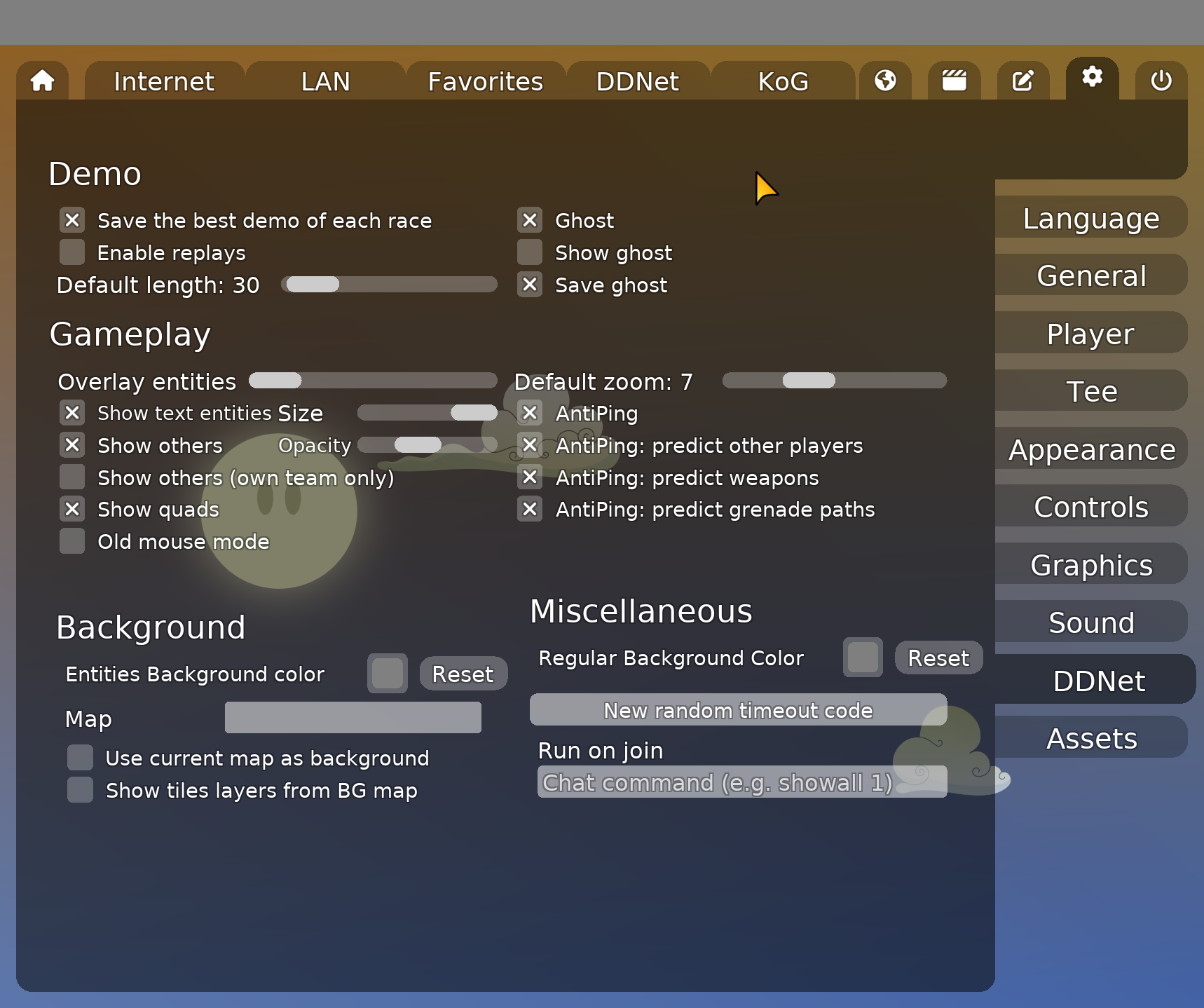
Causes problems with GER3
## Checklist
- [x] Tested the change ingame
- [x] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
5851: Respect reserved slots in old serverinfo r=heinrich5991 a=def-
Noticed in https://github.com/ddnet/ddnet/pull/5850 that reserved slots were not respected
<!-- What is the motivation for the changes of this pull request? -->
<!-- Note that builds and other checks will be run for your change. Don't feel intimidated by failures in some of the checks. If you can't resolve them yourself, experienced devs can also resolve them before merging your pull request. -->
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: def <dennis@felsin9.de>