Seems like there are cases where the texture is not cleared when the MOTD background is rendered, so part of the font texture is used for the round rect.
Main and dummy tee cannot ping each other anymore. Other main and dummy tees can still ping your own main and dummy tees.
We must ensure to only use `m_aLocalIDs[1]` when the dummy is connected, as this ID is not updated when no dummy is connected, so it can refer to another client if you disconnect your dummy and another client subsequently takes its client ID.
Closes#3699.
6439: Refactor `random` functions r=heinrich5991 a=Robyt3
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
On first client start (when `cl_show_welcome` is `1`), determine the user locale with `os_locale_str` and initially select the most fitting language for this locale.
For this the language index must also be loaded immediately on client launch, before the initial language is loaded.
Add `random_float(float min, float max)` to generate a random `float` between `min` and `max`.
Add `random_float(float max)` to generate a random `float` between `0.0f` and `max`.
Replace existing `RandomDir` with this function. This function was biased towards the corners, as it first generates a random `vec2` with `x` and `y` in `[-0.5f;0.5f]` and then normalizes it, which doesn't result in a uniform distribution of directions. Now the random direction is generated by taking a random angle and then converting it to a direction which is per definition already normalized.
To generate a random angle in the range `[0.0f;2.0f * pi[`. This ensures that the random angle cannot be `2.0f * pi`, which would be identical to the angle `0.0f` and therefore cause the random angle to be less uniformly selected.
Note that this first casts `RAND_MAX` to a `float` and then uses `std::nextafter` to get the next larger `float`. Using `RAND_MAX + 1` would cause an integer overflow on systems where `RAND_MAX == INT_MAX` (e.g. Ubuntu and macOS).
When the tee color lighting is set to 0, the blood color was shown as black instead of the actual tee blood color. The blood color used ingame is already correct and now matches the color shown in the settings.
Don't add entered command to console history, if the last console history entry is identical to this command. For example, entering the commands `1`, `2`, `3`, `3`, `2`, `1` adds 5 entries to the history, i.e. only one entry for the command `3`.
Optimize MOTD rendering in ingame menu by caching the text.
Use the correct text height based on the aligned font size instead of the original font size, to fix the discrepancy between the scrollable height and the text height.
Optimize MOTD rendering by caching the round rect and the text.
Align font and rect sizes so exactly 24 lines of text fit in the MOTD rect with margins on all sizes.
6372: Check in CI that no C standard headers are used, replace all usages of C standard headers with C++ headers, replace usages of C math functions r=def- a=Robyt3
Closes#6334.
## Checklist
- [ ] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
Replacing the C standard headers with the C++ standard headers causes various `error: call to 'floor' promotes float to double [performance-type-promotion-in-math-fn,-warnings-as-errors]`, which are fixed by using the C++ std math functions or our own math functions instead of the C math functions.
- Use `absolute` instead of `abs` and `fabs`.
- Use `std::floor` instead of `floor` and `floorf`.
- Use `std::ceil` instead of `ceil`, `ceilf` and `round_ceil`.
- Use `std::round` instead of `round` and `roundf`.
- Use `std::sin` instead of `sin` and `sinf`.
- Use `std::asin` instead of `asin` and `asinf`.
- Use `std::cos` instead of `cos` and `cosf`.
- Use `std::acos` instead of `acos` and `acosf`.
- Use `std::tan` instead of `tan` and `tanf`.
- Use `std::atan` instead of `atan` and `atanf`.
- Use `std::pow` instead of `pow` and `powf`.
- Use `std::log` instead of `log` and `logf`.
- Use `std::log2` instead of `log2` and `log2f`.
- Use `std::log10` instead of `log10` and `log10f`.
- Use `std::pow` instead of `pow` and `powf`.
- Use `std::sqrt` instead of `sqrt` and `sqrtf`.
- Use `std::fmod` instead of `fmod` and `fmodf`.
- Use `direction(Angle)` instead of `vec2(std::cos(Angle), std::sin(Angle))`.
- Use `length(vec2(x, y))` instead of `std::sqrt(x * x + y * y)`.
- Remove unused `NormalizeAngular` and `AngularDistance` functions.
The graphics are not initialised when `RebuildChat` is called by `ConchainChatOld`, which causes the client to crash if `cl_chat_old` is present in the user's config or used as a command line argument.
The maximum width for the server info and game info text was too small, which previously didn't matter, because the manual newlines broke text wrapping in this case, but with the fix from #6353 this now causes the text to break after the colons already.
This is fixed by using the maximum available width for the text instead of a too small magic number (`250.0f`).
For completeness, the maximum width for the title texts is unset (`-1.0f`), because they should never wrap, which is consistent with the MOTD title text.
6351: Fix client crash when echoing client message to chat, use em dash for client messages in chat like on upstream r=def- a=Robyt3
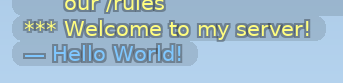
## Checklist
- [X] Tested the change ingame
- [X] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Robert Müller <robytemueller@gmail.com>
Align the color picker selection and reset buttons on the right side instead of the left side dependent on the label width.
Closes#6237.
The `UseCheckBox` argument is removed and instead `pCheckBoxValue != nullptr` is used.
The client crashes when printing a client message to the chat from a config file or from the command line, as the graphics have not been initialized at that point.
Closes#6350.
The `RecreateTextContainer` function calls `DeleteTextContainer` and then `CreateTextContainer`.
The arguments of `RecreateTextContainer` and `RecreateTextContainerSoft` are reordered so all functions take the text container as their first argument.
Reduce duplicate code and improve correctness by passing indices of quad, buffer and text containers by reference and always setting them to `-1` after they are deleted.
Also check if index is `-1` before trying to delete it to reduce duplicate code when calling the methods.
Use `bytes_be_to_uint` and `uint_to_bytes_be` instead.
As casting between `int` and `unsigned` preserves the bit representation of the value, it's not necessary to apply additional tricks to convert between `char` arrays and `int`.
Using `str_format` without format arguments is equivalent to `str_copy`, but using the latter is more efficient and readable.
As `str_format` also returns the result `str_utf8_fix_truncation`, i.e. the potentially truncated string length, the return value is also added to `str_copy` so existing invocations don't need to be adjusted.
6251: Always assume there could potentially be a skin in serverbrowser's pl… r=def- a=Jupeyy
…ayer list
<!-- What is the motivation for the changes of this pull request? -->
<!-- Note that builds and other checks will be run for your change. Don't feel intimidated by failures in some of the checks. If you can't resolve them yourself, experienced devs can also resolve them before merging your pull request. -->
## Checklist
- [x] Tested the change ingame
- [ ] Provided screenshots if it is a visual change
- [ ] Tested in combination with possibly related configuration options
- [ ] Written a unit test (especially base/) or added coverage to integration test
- [ ] Considered possible null pointers and out of bounds array indexing
- [ ] Changed no physics that affect existing maps
- [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional)
Co-authored-by: Jupeyy <jupjopjap@gmail.com>