mirror of
https://github.com/ddnet/ddnet.git
synced 2024-11-19 06:28:19 +00:00
Merge #5756
5756: Editor: added a goto button r=heinrich5991 a=archimede67 <!-- What is the motivation for the changes of this pull request --> This feature was also suggested by Pulsar. It adds a button to go to a specified coordinate point by inputting x and y coordinates through a popup window: 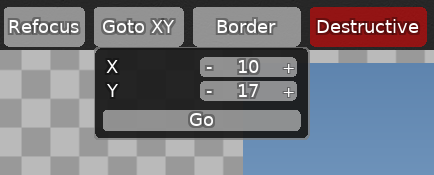 The two number inputs are constrained between 0 and the width/height of the map (minus 1). When clicking "Go", it focuses the camera at the center of the tile at these coordinates. ## Checklist - [x] Tested the change ingame - [x] Provided screenshots if it is a visual change - [ ] Tested in combination with possibly related configuration options - [ ] Written a unit test (especially base/) or added coverage to integration test - [ ] Considered possible null pointers and out of bounds array indexing - [x] Changed no physics that affect existing maps - [ ] Tested the change with [ASan+UBSan or valgrind's memcheck](https://github.com/ddnet/ddnet/#using-addresssanitizer--undefinedbehavioursanitizer-or-valgrinds-memcheck) (optional) Co-authored-by: Corantin H <archi0670@gmail.com>
This commit is contained in:
commit
68e2f51280
|
@ -1095,6 +1095,21 @@ void CEditor::DoToolbar(CUIRect ToolBar)
|
|||
TB_Bottom.VSplitLeft(5.0f, nullptr, &TB_Bottom);
|
||||
}
|
||||
|
||||
// goto xy button
|
||||
{
|
||||
TB_Bottom.VSplitLeft(50.0, &Button, &TB_Bottom);
|
||||
static int s_GotoButton = 0;
|
||||
if(DoButton_Editor(&s_GotoButton, "Goto XY", 0, &Button, 0, "Go to a specified coordinate point on the map"))
|
||||
{
|
||||
static int s_ModifierPopupID = 0;
|
||||
if(!UiPopupExists(&s_ModifierPopupID))
|
||||
{
|
||||
UiInvokePopupMenu(&s_ModifierPopupID, 0, Button.x, Button.y + Button.h, 120, 52, PopupGoto);
|
||||
}
|
||||
}
|
||||
TB_Bottom.VSplitLeft(5.0f, nullptr, &TB_Bottom);
|
||||
}
|
||||
|
||||
// tile manipulation
|
||||
{
|
||||
static int s_BorderBut = 0;
|
||||
|
@ -6064,6 +6079,12 @@ void CEditor::ZoomMouseTarget(float ZoomFactor)
|
|||
m_WorldOffsetY += (Mwy - m_WorldOffsetY) * (1 - ZoomFactor);
|
||||
}
|
||||
|
||||
void CEditor::Goto(float X, float Y)
|
||||
{
|
||||
m_WorldOffsetX = X * 32;
|
||||
m_WorldOffsetY = Y * 32;
|
||||
}
|
||||
|
||||
void CEditorMap::DeleteEnvelope(int Index)
|
||||
{
|
||||
if(Index < 0 || Index >= (int)m_vpEnvelopes.size())
|
||||
|
|
|
@ -835,6 +835,8 @@ public:
|
|||
m_PreventUnusedTilesWasWarned = false;
|
||||
m_AllowPlaceUnusedTiles = 0;
|
||||
m_BrushDrawDestructive = true;
|
||||
m_GotoX = 0;
|
||||
m_GotoY = 0;
|
||||
|
||||
m_Mentions = 0;
|
||||
}
|
||||
|
@ -1239,6 +1241,8 @@ public:
|
|||
static int PopupSpeedup(CEditor *pEditor, CUIRect View, void *pContext);
|
||||
static int PopupSwitch(CEditor *pEditor, CUIRect View, void *pContext);
|
||||
static int PopupTune(CEditor *pEditor, CUIRect View, void *pContext);
|
||||
static int PopupGoto(CEditor *pEditor, CUIRect View, void *pContext);
|
||||
void Goto(float X, float Y);
|
||||
unsigned char m_TeleNumber;
|
||||
|
||||
unsigned char m_TuningNum;
|
||||
|
@ -1249,6 +1253,9 @@ public:
|
|||
|
||||
unsigned char m_SwitchNum;
|
||||
unsigned char m_SwitchDelay;
|
||||
|
||||
int m_GotoX;
|
||||
int m_GotoY;
|
||||
};
|
||||
|
||||
// make sure to inline this function
|
||||
|
|
|
@ -9,6 +9,7 @@
|
|||
#include <engine/keys.h>
|
||||
#include <engine/shared/config.h>
|
||||
#include <engine/storage.h>
|
||||
#include <limits>
|
||||
|
||||
#include <game/client/ui_scrollregion.h>
|
||||
|
||||
|
@ -1698,6 +1699,54 @@ int CEditor::PopupTune(CEditor *pEditor, CUIRect View, void *pContext)
|
|||
return 0;
|
||||
}
|
||||
|
||||
int CEditor::PopupGoto(CEditor *pEditor, CUIRect View, void *pContext)
|
||||
{
|
||||
CUIRect CoordXPicker;
|
||||
CUIRect CoordYPicker;
|
||||
|
||||
View.HSplitMid(&CoordXPicker, &CoordYPicker);
|
||||
CoordXPicker.VSplitRight(2.f, &CoordXPicker, nullptr);
|
||||
|
||||
static ColorRGBA s_Color = ColorRGBA(1, 1, 1, 0.5f);
|
||||
|
||||
enum
|
||||
{
|
||||
PROP_CoordX = 0,
|
||||
PROP_CoordY,
|
||||
NUM_PROPS,
|
||||
};
|
||||
|
||||
CProperty aProps[] = {
|
||||
{"X", pEditor->m_GotoX, PROPTYPE_INT_STEP, std::numeric_limits<int>::min(), std::numeric_limits<int>::max()},
|
||||
{"Y", pEditor->m_GotoY, PROPTYPE_INT_STEP, std::numeric_limits<int>::min(), std::numeric_limits<int>::max()},
|
||||
{nullptr},
|
||||
};
|
||||
|
||||
static int s_aIds[NUM_PROPS] = {0};
|
||||
int NewVal = 0;
|
||||
int Prop = pEditor->DoProperties(&CoordXPicker, aProps, s_aIds, &NewVal, s_Color);
|
||||
|
||||
if(Prop == PROP_CoordX)
|
||||
{
|
||||
pEditor->m_GotoX = NewVal;
|
||||
}
|
||||
else if(Prop == PROP_CoordY)
|
||||
{
|
||||
pEditor->m_GotoY = NewVal;
|
||||
}
|
||||
|
||||
CUIRect Button;
|
||||
View.HSplitBottom(12.0f, &View, &Button);
|
||||
|
||||
static int s_Button;
|
||||
if(pEditor->DoButton_Editor(&s_Button, "Go", 0, &Button, 0, ""))
|
||||
{
|
||||
pEditor->Goto(pEditor->m_GotoX + 0.5f, pEditor->m_GotoY + 0.5f);
|
||||
}
|
||||
|
||||
return 0;
|
||||
}
|
||||
|
||||
int CEditor::PopupColorPicker(CEditor *pEditor, CUIRect View, void *pContext)
|
||||
{
|
||||
CUIRect SVPicker, HuePicker;
|
||||
|
|
Loading…
Reference in a new issue